Auth
Celest comes with built-in auth support via our Cloud Auth (opens in a new tab) package: an open-source, Dart-native service for authenticating and authorizing users in any Dart backend.
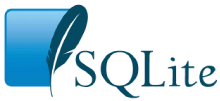
Cloud Auth is built on top of the Cedar (opens in a new tab) policy engine and SQLite (opens in a new tab) to both manage user accounts and enforce consistent access controls in a single Dart package. The Celest CLI will automatically integrate Cloud Auth into your project when using Celest Auth, but the service is exposed as a Shelf router and middleware which can be used in any Dart project.
To get started with Cloud Auth, continue to the next page to see how to add it to your Celest project 🚀
Celest Auth also suppors integrating Supabase and Firebase for cases where you already have an existing user base.